JDK Flight Recorder (JFR) is one of the two prominent open-source profilers for the OpenJDK (besides async-profiler).
It offers many features (see Profiling Talks) and the ability to observe lots of information by recording over one hundred different events.
If you want to know more about the existing events, visit my JFR Event Collection website (related blog post):
Besides these built-in events, JFR allows you to implement your events to record custom information directly in your profiling file.
Let's start with a small example to motivate this. Consider for a moment that we want to run the next big thing after Software-as-a-Service: Math-as-a-Service, a service that provides customers with the freshest Fibonacci numbers and more.
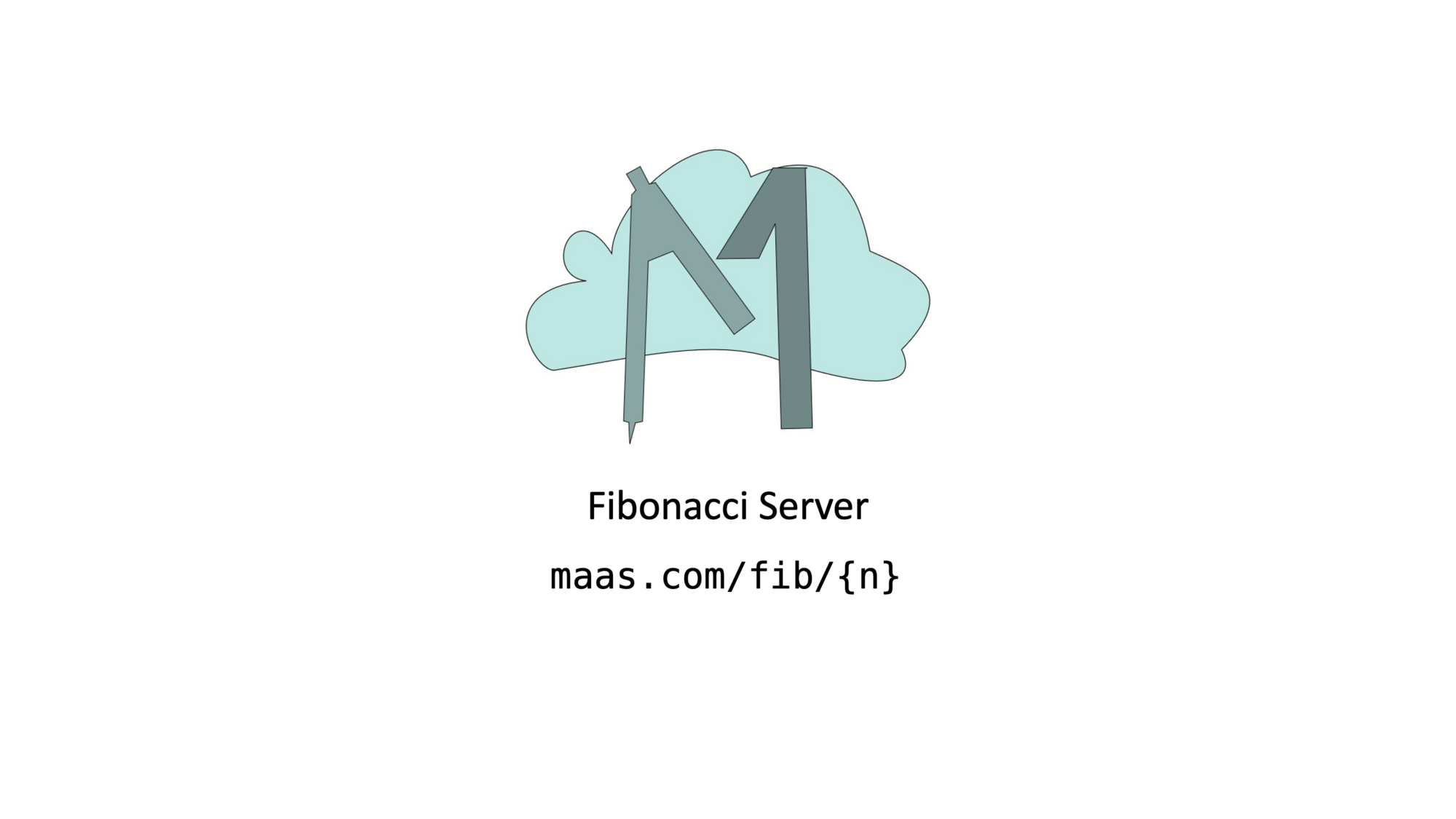
We develop this service using Javalin:
public static void main(String[] args) throws Exception { // create a server with 4 threads in the thread pool Javalin.create(conf -> { conf.jetty.server(() -> new Server(new QueuedThreadPool(4)) ); }) .get("/fib/{fib}", ctx -> { handleRequest(ctx, newSessionId()); }) .start(7070); System.in.read(); } static void handleRequest(Context ctx, int sessionId) { int n = Integer.parseInt(ctx.pathParam("fib")); // log the current session and n System.out.printf("Handle session %d n = %d\n", sessionId, n); // compute and return the n-th fibonacci number ctx.result("fibonacci: " + fib(n)); } public static int fib(int n) { if (n <= 1) { return n; } return fib(n - 1) + fib(n - 2); }
This is a pretty standard tiny web endpoint, minus all the user and session handling. It lets the customer query the n-th Fibonacci number by querying /fib/{n}.
Our built-in logging prints n and the session ID on standard out, but what if we want to store it directly in our JFR profile while continuously profiling our application?
This is where custom JFR events come in handy:
public class SessionEvent extends jdk.jfr.Event { int sessionId; int n; public SessionEvent(int sessionId, int n) { this.sessionId = sessionId; this.n = n; } }
The custom event class extends the jdk.jfr.Event
class and simply define a few fields for the custom data. These fields can be annotated with @Label("Human readable label")
and @Description("Longer description")
to document them.
We can now use this event class to record the relevant data in the handleRequest
method:
static void handleRequest(Context ctx, int sessionId) { int n = Integer.parseInt(ctx.pathParam("fib")); System.out.printf("Handle session %d n = %d\n", sessionId, n); // create event var event = new SessionEvent(sessionId, n); // add start and stacktrace event.begin(); ctx.result("fibonacci: " + fib(n)); // add end and store event.commit(); }
This small addition records the timing and duration of each request, as well as n
and the session ID in the JFR profile. The sample code, including a request generator, can be found on GitHub.
After we ran the server, we can view the recorded events in a JFR viewer, like JDK Mission Control or my JFR viewer (online view):
This was my short introduction to custom JFR events; if you want to learn more, I highly recommend Gunnar Morlings Monitoring REST APIs with Custom JDK Flight Recorder Events article.
Come back next week for a real-world example of custom JFR events.
This article is part of my work in the SapMachine team at SAP, making profiling and debugging easier for everyone. It first appeared on my personal blog mostlynerdless.de.