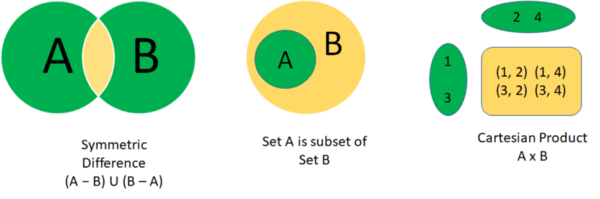
Primitive Set Operations in Eclipse Collections - Part 2
Continuing from Part 1, in the Eclipse Collections 11.0 release, we will have the following Set operations union
, intersect
, difference
, symmetricDifference
, isSubsetOf
, isProperSubsetOf
, cartesianProduct
on primitive collections.
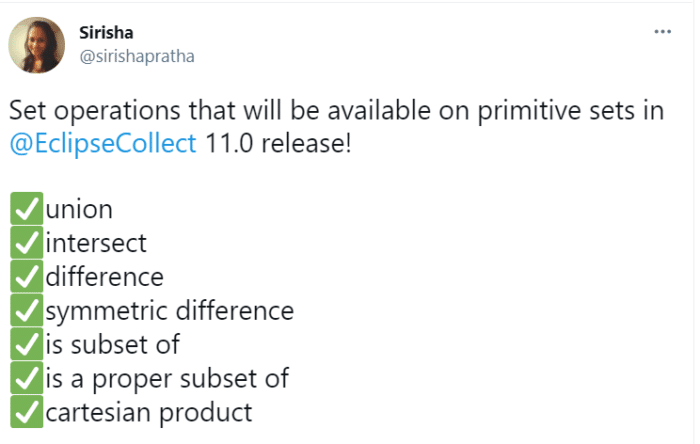
Set Operations that will be available on primitive sets in Eclipse Collections 11.0.
These APIs are already available on Sets on the object side, but my goal was to implement these methods for Sets supporting primitive types namely IntSet
, LongSet
, ShortSet
, DoubleSet
, FloatSet
, CharSet
, BooleanSet
, ByteSet
. Additionally, the above operations will be available in both mutable and immutable interfaces.
I covered the first three operations (union
, intersect
and difference
) in this blog. I will talk about the remaining operations here.
Symmetric Difference — What does this operation do?
Method signature: setA.symmetricDifference(setB)
This operation returns a set that contains elements that are in either one of the two sets but not both. In other words, (Set A — Set B) U (Set B — Set A).
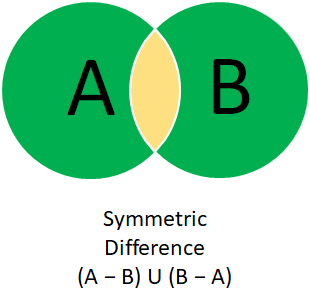
Set B — 2, 3, 4.
A — B = 1.
B — A= 4.
Symmetric Difference — 1, 4.
Symmetric Difference — Code Implementation
🛈 Eclipse Collections has an existing API that allows us to reject
that returns all the elements that evaluate false for the givenPredicate
and results are added to a target collection. Predicate here is this::contains
and target collection is the set returned from this.difference(set)
.
At this point of implementing symmetricDifference
, we already had an API that can compute difference
of Set A and Set B.
default MutableIntSet symmetricDifference(IntSet set) { return set.reject(this::contains, this.difference(set)); }
Symmetric Difference — Usage
@Test public void symmetricDifference() { MutableIntSet setA = IntSets.mutable.with(1, 2, 3); MutableIntSet setB = IntSets.mutable.with(2, 3, 4); MutableIntSet expected = IntSets.mutable.with(1, 4); MutableIntSet actual = setA.symmetricDifference(setB); Assert.assertEquals(expected, actual); }
Test cases covering various scenarios can be found here.
Comments (0)
No comments yet. Be the first.