Aspect-oriented programming (AOP) is a programming paradigm that seeks to increase modularity by separating cross-cutting concerns from core application logic.
Cross-cutting concerns refer to functionality like logging, security, and transactions that span across multiple areas of an application.
AOP allows these concerns to be encapsulated in reusable modules called aspects.
This improves modularity and makes the codebase easier to maintain.
In large-scale Java applications, AOP is invaluable for avoiding code duplication and keeping the core code focused on business goals.
Understanding Cross-Cutting Concerns
Cross-cutting concerns are features or behaviors spread across multiple locations in code. Some examples include:
-
Logging 📝 - Tracing information spread across many classes and methods
-
Security 🔐- Authentication and authorization logic required in multiple places
-
Caching 🔄- Caching logic duplicated in many classes
Traditional object-oriented code leads to code duplication and tight coupling when implementing these kinds of concerns. AOP solves this by providing means to modularize cross-cutting concerns into cohesive units.
Core Concepts of AOP 🛠️
The main concepts in AOP are:
Aspect: A module containing advice and pointcuts related to a cross-cutting concern. For example, a logging aspect.
Join point: A point in program execution where advice can be applied. This is usually a method invocation.
Advice: Code to execute at a join point. There are different types of advice:
- Before advice: Run before the join point
- After advice: Run after the join point
- Around advice: Run before and after the join point
Pointcut: An expression that selects join points where advice should run. Pointcuts use expressions based on method names, annotations, object types, etc.
Weaving: The process of applying advice at join points selected by pointcuts. It is done by the AOP framework.
AOP in Java: The AspectJ Framework 🌐
The most widely used AOP framework for Java is AspectJ. It extends Java with language constructs for implementing aspects and pointcuts directly in code. AspectJ supports compile-time and runtime weaving. Some key features are:
-
@Aspect annotation for defining aspects
-
Pointcut expressions like "execution()"
-
Advice types - @Before, @After, @Around
AspectJ requires some setup in the build configuration and IDE. However once configured, it streamlines implementing aspects in a Java project.
Here's a simple logging aspect using AspectJ:
import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.aspectj.lang.annotation.After; import org.aspectj.lang.JoinPoint; import org.aspectj.lang.annotation.Pointcut; import org.springframework.stereotype.Component; @Aspect @Component public class LoggingAspect { // Pointcut for all methods in the service package @Pointcut("execution(* com.healthcare.service.*.*(..))") public void serviceLayer() {} // Before advice for logging @Before("serviceLayer()") public void logBefore(JoinPoint joinPoint) { System.out.println("Entering Method: " + joinPoint.getSignature().getName() + " in " + joinPoint.getTarget().getClass().getSimpleName()); } // After advice for logging @After("serviceLayer()") public void logAfter(JoinPoint joinPoint) { System.out.println("Exiting Method: " + joinPoint.getSignature().getName() + " in " + joinPoint.getTarget().getClass().getSimpleName()); } }
The pointcut expression identifies the join points (method executions) where advice will be applied.
Benefits of AOP ✨
Some key benefits of using AOP:
-
Avoid code duplication by encapsulating cross-cutting concerns
-
Core code focuses on business goals rather than utilities
-
Easier to modify or add features like logging
-
Increased modularity for improved maintenance specially in Microservices Architecture which you call learn more in the following article Microservices Introduction
AOP allows clean separation of concerns in Java code, enhancing readability and maintainability. By encapsulating cross-cutting behaviors in aspects, duplication is eliminated leading to more cohesive and focused code.
Conclusion 📚
Aspect-oriented programming enables better separation of concerns in Java applications through the use of aspects and pointcuts.
The AspectJ framework streamlines AOP adoption in Java. AOP improves modularity which leads to code that is easier to understand and maintain.
In the upcoming article we will see the AOP in Action, stay tuned.
References 📖
Quick SQL Query Optimization
Identify the root cause of a slow SQL query and optimize it in a fraction of the time it could have taken you.
See how!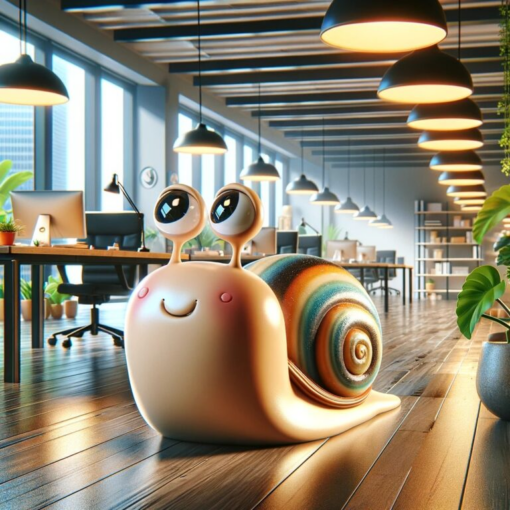
[…] >> Aspect-Oriented Programming (AOP) Made Easy With Java [foojay.io] […]